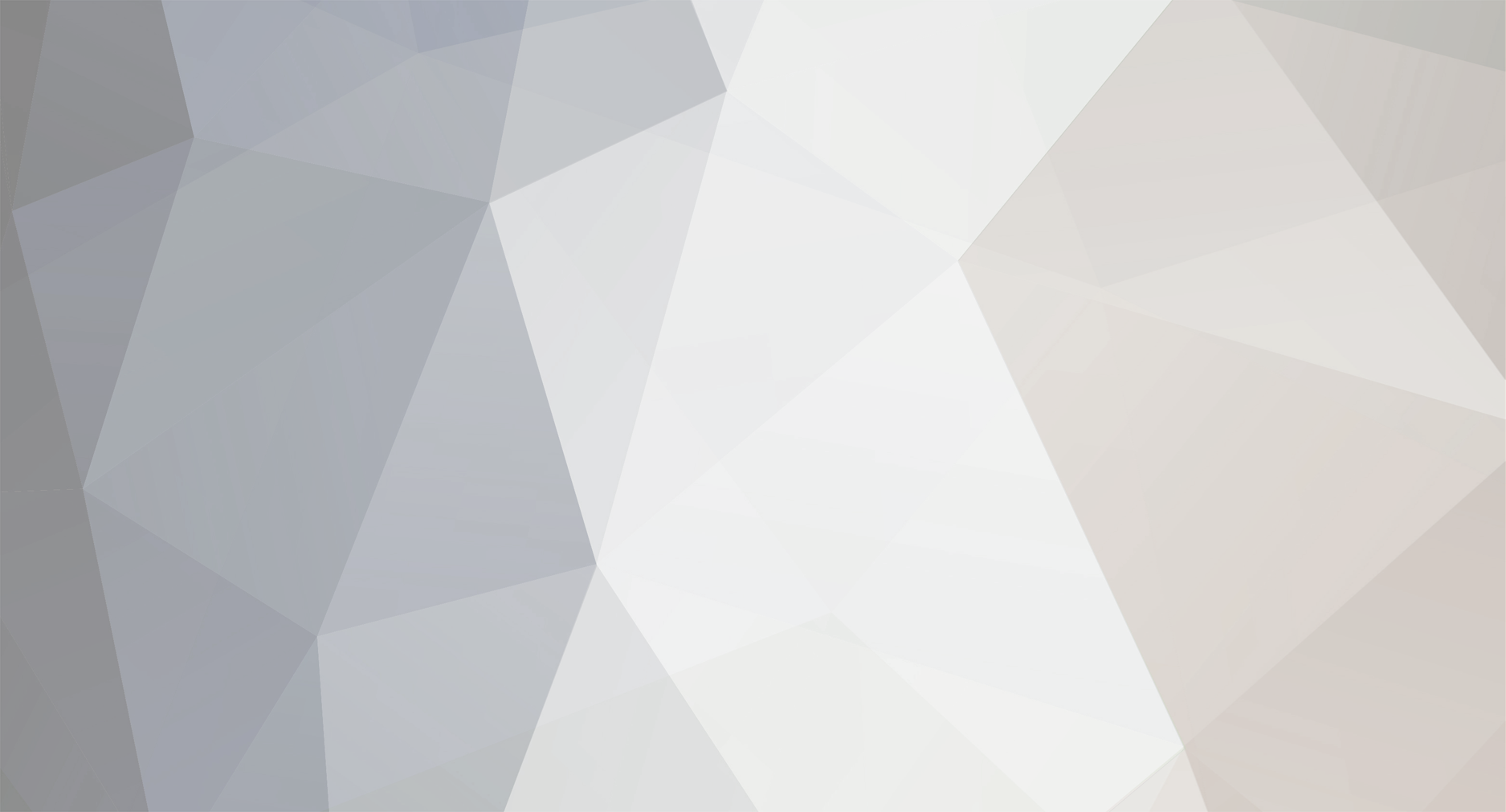
deadeye
-
Posts
24 -
Joined
-
Last visited
Content Type
Profiles
Forums
Articles
Posts posted by deadeye
-
-
It looks like you're selecting one random item as soon as your array is created and that's the only item you use from that array.
Here's what I think you want to do:
Remove thecall BIS_fnc_selectRandom
from the end of your arrays and then add your loot with something like this:
_this addMagazineCargoGlobal [(_loot_build call BIS_fnc_selectRandom),(10 + floor(random 2))]; _this addMagazineCargoGlobal [(_loot_build call BIS_fnc_selectRandom),(10 + floor(random 2))]; ...
Also, in _crate_4 you're adding _loot_clothes and I think it should be _loot_uniforms
-
There's already a line in the code to not allow changing clothes while wearing a backpack but it has been commented out. Try this:
In your custom compiles.sqf, put this line
player_wearClothes = compile preprocessFileLineNumbers "custom\player_wearClothes.sqf";
Then create this file in your custom folder and name it player_wearClothes.sqf
Line 24 is the only one that was changed from the default file.
-
Try something like this:
if (_isMine) then { _rnd = random 100; if (_rnd < 40) then {_gem = "ItemTopaz";}; //13.6% if (_rnd < 26.4) then {_gem = "ItemObsidian";}; //9.2% if (_rnd < 17.2) then {_gem = "ItemSapphire";}; //6.4% if (_rnd < 10.8) then {_gem = "ItemAmethyst";}; //4.4% if (_rnd < 6.4) then {_gem = "ItemEmerald";}; //3.2% if (_rnd < 3.2) then {_gem = "ItemCitrine";}; //2% if (_rnd < 1.2) then {_gem = "ItemRuby";}; //1.2% _selectedRemoveOutput set [(count _selectedRemoveOutput),[_gem,1]]; };
-
You use a function called BIS_fnc_infoText
One example is in dayz_code/system/player_spawn_1.sqf
Also see:
http://www.ofpec.com/COMREF/index.php?action=read&id=231#infotext
-
The line in your custom compiles.sqf should point to the location of your customized remove.sqf file, like this:
player_removeObject = compile preprocessFileLineNumbers "scripts\remove.sqf";
-
Not directly but it includes its own copy of selfActions. Is that the one you modified?
I know you mentioned you didn't see any errors in the RPT, is that in both server and client RPT files?
-
Yes, as long as maintain_area.sqf is in your custom folder.
The section of your fn_selfActions.sqf should look like this:
if (_canDo && (speed player <= 1) && (_cursorTarget isKindOf "Plastic_Pole_EP1_DZ")) then { if (s_player_maintain_area < 0) then { s_player_maintain_area = player addAction [format["<t color='#ff0000'>%1</t>",localize "STR_EPOCH_ACTIONS_MAINTAREA"], "custom\maintain_area.sqf", "maintain", 5, false]; s_player_maintain_area_preview = player addAction [format["<t color='#ff0000'>%1</t>",localize "STR_EPOCH_ACTIONS_MAINTPREV"], "custom\maintain_area.sqf", "preview", 5, false]; }; } else { player removeAction s_player_maintain_area; s_player_maintain_area = -1; player removeAction s_player_maintain_area_preview; s_player_maintain_area_preview = -1; };
Sorry, it has worked flawlessly for me.
-
Did you change the lines in your fn_selfActions to point to the correct location?
-
I hope it becomes standard with the next version of Epoch.
I only briefly tested it. Let me know how it works for you and I'll make a pull request.
-
This works for me (with limited testing):
Create a file in your scripts folder called maintain_area.sqf with this code:
//Code developed by Axe Cop - Massiv improvments && performance tunes by Skaronator - simplified by Deadeye private ["_textMissing","_target","_objectClasses","_range","_objects","_requirements","_count","_cost","_itemText","_option","_objects_filtered"]; if (DZE_ActionInProgress) exitWith { cutText [(localize "STR_EPOCH_ACTIONS_2") , "PLAIN DOWN"]; }; DZE_ActionInProgress = true; player removeAction s_player_maintain_area; s_player_maintain_area = 1; player removeAction s_player_maintain_area_preview; s_player_maintain_area_preview = 1; _target = cursorTarget; // Plastic_Pole_EP1_DZ _objectClasses = DZE_maintainClasses; _range = DZE_maintainRange; // set the max range for the maintain area _objects = nearestObjects [_target, _objectClasses, _range]; //filter to only those that have 10% damage _objects_filtered = []; { if (damage _x >= DZE_DamageBeforeMaint) then { _objects_filtered set [count _objects_filtered, _x]; }; } count _objects; _objects = _objects_filtered; // TODO dynamic requirements based on used building parts? _count = count _objects; if (_count == 0) exitWith { cutText [format[(localize "STR_EPOCH_ACTIONS_22"), _count], "PLAIN DOWN"]; DZE_ActionInProgress = false; s_player_maintain_area = -1; s_player_maintain_area_preview = -1; }; _requirements = []; switch true do { case (_count <= 10): {_requirements = ["ItemGoldBar10oz",1]}; case (_count <= 20): {_requirements = ["ItemGoldBar10oz",2]}; case (_count <= 35): {_requirements = ["ItemGoldBar10oz",3]}; case (_count <= 50): {_requirements = ["ItemGoldBar10oz",4]}; case (_count <= 75): {_requirements = ["ItemGoldBar10oz",6]}; case (_count <= 100): {_requirements = ["ItemBriefcase100oz",1]}; case (_count <= 175): {_requirements = ["ItemBriefcase100oz",2]}; case (_count <= 250): {_requirements = ["ItemBriefcase100oz",3]}; case (_count <= 325): {_requirements = ["ItemBriefcase100oz",4]}; case (_count <= 400): {_requirements = ["ItemBriefcase100oz",5]}; case (_count <= 475): {_requirements = ["ItemBriefcase100oz",6]}; case (_count <= 550): {_requirements = ["ItemBriefcase100oz",7]}; case (_count <= 625): {_requirements = ["ItemBriefcase100oz",8]}; case (_count > 625): {_requirements = ["ItemBriefcase100oz",9]}; }; _option = _this select 3; switch _option do { case "maintain": { if !([[_requirements],0] call epoch_returnChange) then { _textMissing = getText(configFile >> "CfgMagazines" >> _requirements select 0 >> "displayName"); cutText [format[(localize "STR_EPOCH_ACTIONS_6"), _requirements select 1, _textMissing], "PLAIN DOWN"]; } else { player playActionNow "Medic"; [player,_range,true,(getPosATL player)] spawn player_alertZombies; cutText [format[(localize "STR_EPOCH_ACTIONS_4"), _count], "PLAIN DOWN", 5]; PVDZE_maintainArea = [player,1,_target]; publicVariableServer "PVDZE_maintainArea"; }; }; case "preview": { //_cost = ""; _itemText = getText(configFile >> "CfgMagazines" >> _requirements select 0 >> "displayName"); _cost = str(_requirements select 1) + " of " + _itemText; cutText [format[(localize "STR_EPOCH_ACTIONS_7"), _count, _cost], "PLAIN DOWN"]; }; }; DZE_ActionInProgress = false; s_player_maintain_area = -1; s_player_maintain_area_preview = -1;
Then in your fn_selfActions.sqf:
changes_player_maintain_area = player addAction [format["<t color='#ff0000'>%1</t>",localize "STR_EPOCH_ACTIONS_MAINTAREA"], "\z\addons\dayz_code\actions\maintain_area.sqf", "maintain", 5, false]; s_player_maintain_area_preview = player addAction [format["<t color='#ff0000'>%1</t>",localize "STR_EPOCH_ACTIONS_MAINTPREV"], "\z\addons\dayz_code\actions\maintain_area.sqf", "preview", 5, false];
to
s_player_maintain_area = player addAction [format["<t color='#ff0000'>%1</t>",localize "STR_EPOCH_ACTIONS_MAINTAREA"], "scripts\maintain_area.sqf", "maintain", 5, false]; s_player_maintain_area_preview = player addAction [format["<t color='#ff0000'>%1</t>",localize "STR_EPOCH_ACTIONS_MAINTPREV"], "scripts\maintain_area.sqf", "preview", 5, false];
If you put it somewhere other than your scripts folder, change it to match the location.
-
Dunno exactly, but isnt floor(random 3) not like 0,1,2?
Yes, because random x returns a floating point number from 0 (inclusive) to x (not inclusive)
https://community.bistudio.com/wiki/random
(random 3) might return 2.99999 but never 3
-
-
Each one of your strings in the format command has to have a %1 before the closing quote.
systemChat(format["Classname: %1",_typeOfCursorTarget]);
-
anyone got a way to implement the payment functionality the traders have?
in the files:
service_point_rearm.sqf
service_point_refuel.sqf
service_point_repair.sqf
find the line near the top of each file that reads:
if !([_costs] call player_checkAndRemoveItems) exitWith {};
and replace it with this:
if !([[[_costs select 0, _costs select 1]],0] call epoch_returnChange) exitWith {};
- Cinjun, OneManGang and Axe Cop
-
3
-
Im guessing the code checking if a tank is near is faulty.
Correct. It says 30 but it's actually looking within 3 meters.
Fixed in my pull request:
https://github.com/vbawol/DayZ-Epoch/commit/be3cc5e5a5d95df857df4175a596a3fdf9ddce4c
-
-
Are you using DayZ.st?
If so, check this:
-
-
Could'nt clear Major Mission 10
Mission 10 looks okay. Do you mean major mission 7?
-
mission8 the pbx one wont finish? couldnt find the tent that was ment to spawn any help would be awesome thanks
Sometimes we can swim out to the boat and pilot it to shore, then the tent will appear and the mission will clear.
-
These errors:
10:24:25 Error in expression <loadout select 2; _aiammo2 = _ailoadout select 3; _aiunit addweapon _aiwep1; _ai> 10:24:25 Error position: <select 3; _aiunit addweapon _aiwep1; _ai> 10:24:25 Error Zero divisor
should be fixed by my github commit here.
-
-
In Missions/Minor/SM10.sqf lne 29 at the end is:
0.01, ];
shouldn't it be
0.01 ];
[RELEASE] Random Loot Crates v1.25
in Scripts
Posted
try this: