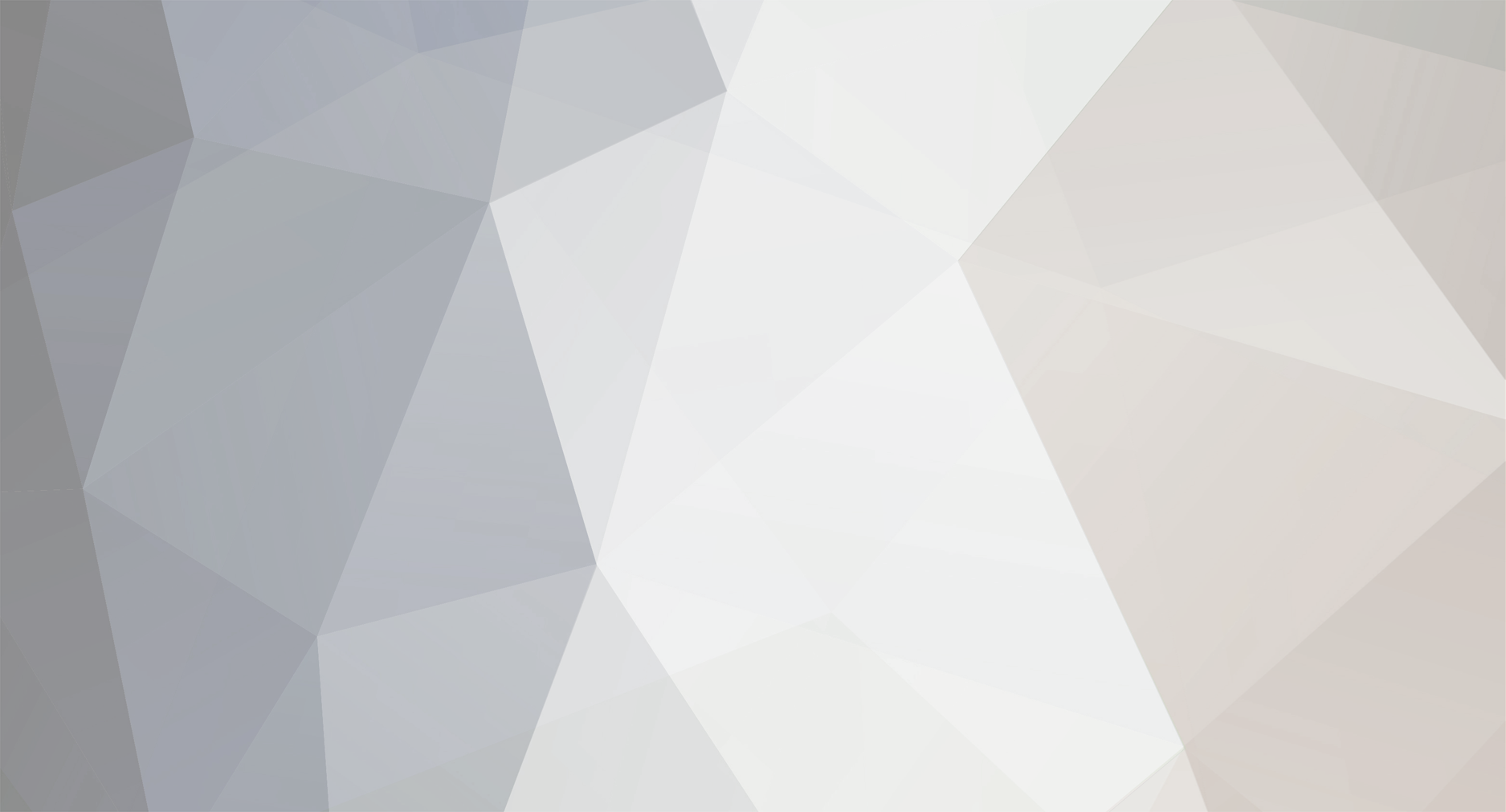
Leigham
-
Posts
322 -
Joined
-
Last visited
-
Days Won
1
Content Type
Profiles
Forums
Articles
Posts posted by Leigham
-
-
Thanks for letting me know :)
-
does anyone if this works with the new version, if it doesnt I might update it :D
-
Still need help if poss
-
plenty of ways to do it. One way is storing the variable somewhere, like a global variable, or send it back via function, like so:
funcion_amountOfApples = { _apples = 10; //set or calculate stuffs here _apples //return value }; //now we have a function that returns a value, here's how we retrieve it: //create variable with value gathered from function above _appleCount = call funcion_amountOfApples; hint str _appleCount; //10
So essentially, you can process data in a loop, store it in some variable and either save it into global variable (not the best idea), or return it to a caller. Caller can then define a new local/global variable from data gathered.
Functions 101, really.
And ofc you can also send information to functions and ask for value in return:
//create function _someFunction = { _input = _this; //collect data from input _fruit = _input select 0; _amount = _input select 1; //add random amount of fruits and return the result to caller _return = _amount + round random 5; _return }; //now lets call it _addFruits = ["apples",10] call _someFunction; hint str _addFruits; //number between 10 and 15
_GetVehicleClassnameFromList = { { if(_selectionData == (_x select 0))then{ _VehicleClassname = _x Select 0; diag_log format ["%1",_VehicleClassname]; // Gives the Right Classname; }; }foreach UG_VehicleList; _VehicleClassname // Return Variable. // Is this right, in this position ? }; _Vehicleinfo = call _GetVehicleClassnameFromList; // Calling the function to get the classname from the list. Diag_Log format ["%1",_VehicleInfo]; //This One, Undefined Variable.
This is what Ive put to first test what you have given me and alter in the way I think I need to.
-
Still Stuck on this part. Any help is great.
-
So Basically, the best way to do it for me would be, one function to get the wanted array, and sort them into variables. then another function for each variable, that needs one, (IE. one for checking the inventory, one for the spawning of the crafted vehicle, and removing items, an such
Edit,
Something like if the classname was "Mosquito_Epoch" _GetChosenVehicleClassname = { if(_selectionData == (_x select 0))then{ _VehicleClassname = _x Select 0; _VehicleClassname }; }foreach UG_VehicleList; _VehicleClassname = Call _GetChosenVehiclename; Systemchat format ["%1",_VehicleClassname]; Would that return "Mosquito_Epoch" ?
-
I Dont know whats going on, im not too sure on how to pass a variable out of the foreach loop, to be used outside of it after searching for the Items heres what I have
disableSerialization; _display = findDisplay 7770; _ObjectTypeText = _display displayCtrl 7779; _typeselection = lbCurSel _ObjectTypeText; _ObjectTypeName = lbtext [7779,_typeselection]; _index = lbCurSel _ObjectTypeText; //index is required to access data _selectionData = _ObjectTypeText lbData _index; //className we saved earlier in a data field diag_log format ["%1-%2",_selectionData,_ObjectTypeName]; { if(_selectionData == (_x select 0))then{ Diag_Log format ["%1",_x];//Dialogging the Whole Chosen Array. _CraftingItems = _x Select 1;//Getting the Items Needed to Craft _CraftingSave = _x Select 2;//Is the Vehicle going to be saved ? _CraftingKrypto = _x Select 3;//How Much Krypto _CraftingIteems pushBack [_craftingItems]; //Arma 3 }; _itemsOnPlayer = (magazines player) + (items player) + (weapons player); _found = []; _foundPlusCount = []; //Find and Find + count { if (_x in _itemsOnPlayer) then { //found item on player if !(_x in _found) then { //but only if item is not already added _found pushBack _x; //Arma 3 _y = _x; //can't use magic var further, so store it in _y or w/e _count = { _x == _y} count _itemsOnPlayer; _foundPlusCount pushBack [_y,_count]; //Arma 3 systemchat format ["%1 %2,_Count,_foundPlusCount"]; }; }; } forEach _CraftingIteems; }forEach UG_VehicleList;
-
This is where im up too
Say I wanted to search a players inventory for a random array of items
ThisNestedArrays = [ ["ItemClassname",[1,1,1,2,2,3]], ["ItemClassname",[2,3,3,4,5]], ["ItemClassname",[2,4,5,6,7,7,3]] ]; I know i would use a foreach loop to check something like, { _ItemClassname = _x select 0; _ItemArray = _x Select 1; //this is the part I need help with //Something like { if(_x in (magazines player))then{ //Maybe ? I want it to check for all the Items in the players inventory before carrying on. }foreach _ItemArray; }foreach ThisNestedArrays;
Any Help would be Great, Can provide a Github, link for people who want a look.
Edit, forgot to put, each number represents an Item, and the multiples are there for a reason, so if I needed to search for a multiple of the same Item.
-
Streaming this now if anyone wants to take a look, see what im doing wrong xD
-
So Im to the point where it is checking the players inventory for the required Items and got it to Diag _log what is needed, but if there is more than 1 of an Item, it is still only checking if there is 1 in his inventory.
-
The list box is showing the Display name already, im onto the actual spawning part of the dialog, after clicking the spawn button. just need to find a way of searching for multiples of the same item.
UG_Classname Vehicle is changing the displayname to classname, im using that to changed the selected item into the Classname, so I can compare that to the {_x select 0;}foreach UG_VehicleList; in the spawn vehicle script.
-
https://github.com/Leigham/Epoch-Action-Menu/blob/master/ActionMenu/Scripts/SpawnVehicle.sqf specifically this file. if you could take a look it would be great.
-
Im working on a script, and Im so Close to finishing, just this one thing Keeps getting stuck Ill explain Below.
If I have an array.
UG_VehicleList = [ ["Mosquito_Epoch",[""],false,0], ["Mosquito_Epoch",[""],true,0], ["Ebike_Epoch",[""],false,0], ["Ebike_Epoch",[""],true,0] ];
and I wanted to do
if(_CraftingItems == "")then{exitwith {};}else{ { _CraftingItems = _x select 2; if(_CraftingItems in (magazines player))then{ }foreach UG_VehicleList; }
I cant get that to check for multiples of the same item, I want it to check if the player has all the items in the array before it takes it out.
-
For the Crafting part of it, I have an array, which you can add more vehicles to it.
["Mosquito_Epoch",[""],true,0]
["Vehicle_Classname",["Items It Will Cost"],SavetoDB true or false,how much krypto it will cost]
-
Before doing do, Could I Maybe Implement this into my actionmenu ?
-
In Progress of updating now.
-
Now got this issue sorted but adding it to a server nothing works :(
-
ive been working on something for a while now, and its come to this problem
_holder = “WeaponHoldersimulated” createVehicle position player;_holder addbackpackcargoglobal [“_classname”,_amounttospawn];
diag_log format [“%1 %2″,_Classname, _AmounttoSpawn];the log is coming up with what it should.
“B_Carryall_cbr 1″but no backpack,
it is working in editor, i can spam and get surrounded in backpacks, but not working through the script.
vehicles are spawning fine, but the "groundweaponholder" is not
-
I could take a look, maybe put an option in if the vehicle is in the config traders then get the price out of there. ill have to take a look.
-
I get this error when i start the server
ErrorMessage: File mpmissions\__cur_mp.Chernarus\custom\keymaker\keymaker.hpp, line 5: /KeyMakerLega/controlsBackground.Lega_BG_Main: Undefined base class 'IGUIBACK'
This is with the updated version.
thx again for this script :) hope i can figure out the problem
have you done the defines step ?
-
Updated with new instructions on github.
-
Ah nice. Few things to make it work better (the scroll menu kept flashing so fixed):
Key_Maker = ["USMC_SoldierS_SniperH"]; //skin of choice - to variables.sqf
In fn_selfactions.sqf add this as alternative to the add action you have:
*define s_maker_dialog = -1; at the bottom of fn_self actions and in the variables.sqf
Also, your close option comes up with error missing ; fixed by going to KeyMaker.hpp go to exit button and change to:
onbuttonclick = "((ctrlParent (_this select 0)) closeDisplay 7459);";
Hope this helped
Max
Will take a look now, and update the tut accordingly
-
I was trying to add it to the scroll but kept getting unknown attribute side. still working on that one.will be updating later.
-
[Release] KeyMaker
in A2: Epoch Mods (1.0.5.1)
Posted
since making this I have come a little way way it comes to developing, so in the next few days, I will put up a test server, and remake this :)