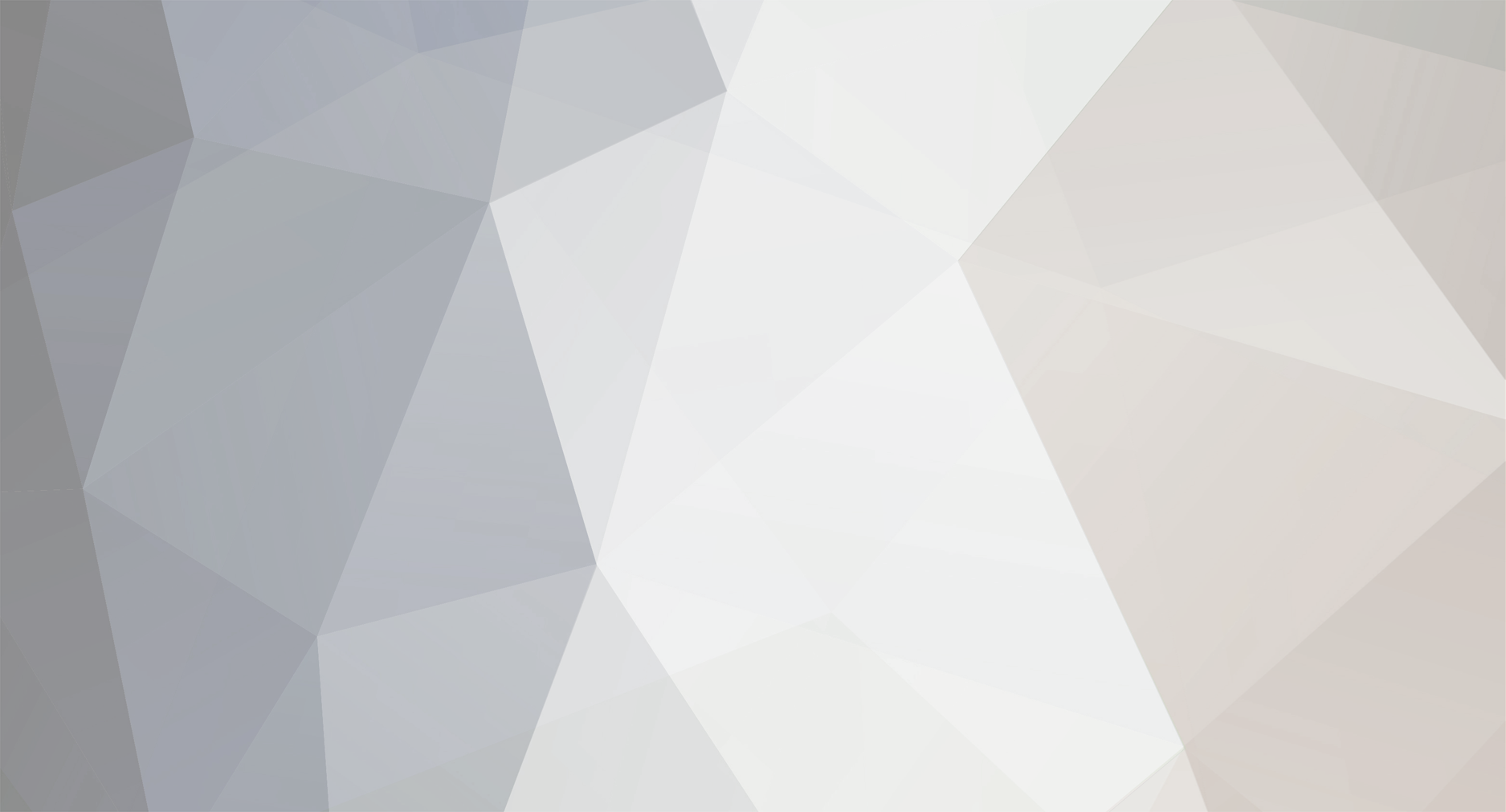
hambeast
-
Posts
462 -
Joined
-
Last visited
-
Days Won
5
Content Type
Profiles
Forums
Articles
Posts posted by hambeast
-
-
interesting.... I think I have an old description.ext then. Thanks guys!
-
Hey all,
I did a search and only found marginally helpful information. Does anyone know off the top of their head how to add an area to the map that is a no-build zone?
Figured I'd ask before digging deep into the client/server code if somebody had figured this out already.
-
And so more info on that, it is instantaneous when you edit their account from Redis
there's a post I made on how to make changes to the banks in game programatically.
Here's how it works... mostly.
1. Player logs in (client has no idea of bank balance, it is null now)
2. Player opens bank terminal at payphone (bank balance is loaded)
3. Player does something with bank (bank balance altered)
4. Player closes bank windows (bank balance is nulled again on clientside)
so you need to emulate step #2 to grab their balance and then pass the right hive calls to modify their balance.
an in answer to your previous question, so long as the player does not have their bank terminal open, any changes to their redis balance is instant.
-
It seems having AIA/Bornholm FIRST in the mods command line breaks shit...
that was it!
-
Having issues updating my dev server this afternoon. Players get stuck here:
-
22:51:57 Mission file: Epoch_Intro
-
22:51:57 Mission world: Altis
-
22:51:57 Mission directory: x\addons\a3_epoch_config\scenes\Epoch_Intro.Altis\
I'm running chernarus too... so IDK what that is about...
-
-
Confirmed, seeing this as well on 0.3.0.1
-
I... don't think so. You could try turning the screen red like the cultists do?
-
Thanks again hambeast for the scripting help. I'm not all that familiar with sqf, but I recognize coding patterns from other languages.
I had to add in a wait for ingame and I did some other modifications to the script.
With your permission I'd like to post the final script in the A3 Epoch Resources/Scripts for others to use.
Original script credit to you of course.
Here is the final script I intend to post.
//ranks.sqf //Original Script by hambeast //Modified and Completed by Evan Black // Wait for ingame waitUntil {!isNuLL(uiNameSpace getVariable ["EPOCH_loadingScreen",displayNull])}; waitUntil {isNuLL(uiNameSpace getVariable ["EPOCH_loadingScreen",displayNull])}; sleep 2; //Optional delay to ensure script execution // Rank/UID list. Use Example Below. //UID Example: Ranks_CORPORAL = ["7656xxxxxxxxxxxxxx","7656xxxxxxxxxxxxxx"]; no trailing coma Ranks_CORPORAL = []; Ranks_SERGEANT = []; Ranks_LIEUTENANT = []; Ranks_CAPTAIN = []; Ranks_MAJOR = []; Ranks_COLONEL = []; _playerUID = getPlayerUID player; //get player UID // loop to check to see if our player matches _found = false; while {!_found} do { if (_playerUID in Ranks_CORPORAL) then { player setRank "CORPORAL"; _found = true; // endloop }else{ if (_playerUID in Ranks_SERGEANT) then { player setRank "SERGEANT"; _found = true; }else{ if (_playerUID in Ranks_LIEUTENANT) then { player setRank "LIEUTENANT"; _found = true; }else{ if (_playerUID in Ranks_CAPTAIN) then { player setRank "CAPTAIN"; _found = true; }else{ if (_playerUID in Ranks_MAJOR) then { player setRank "MAJOR"; _found = true; }else{ if (_playerUID in Ranks_COLONEL) then { player setRank "COLONEL"; _found = true; }else{ player setRank "PRIVATE"; //setting default rank for all others _found = true; }; }; }; }; }; }; };
Swash, I am so happy what I typed helped you out! I think we forget sometimes, just how hard it is to make the jump into Arma coding from other languages. But... once you get the basics, the rest will become clear.
-
first google result:
https://community.bistudio.com/wiki/setRank
You would call this within your script block.
player setRank "COLONEL"
If you're asking how to implement this in your mission file... You could hardcode your group members ranks by ID in your init.sqf or anywhere else in your mission file if you liked...
something like this could work... no idea if it actually does what you want though...
// ranks.sqf - called from init.sqf // we could have 1 big multi-dim array containing each users ID and Rank but... // that would make this harder to adjust in the future since this is a manual // process. If you were to load this data from a DB for instance, you might // want to keep it in a multi-dimensional array like: // [["1234","PRIVATE"],["231","General"]] Ranks_PRIVATE = [ "1234567", // Me "7654321" // No trailing ,! ]; Ranks_CORPORAL = []; Ranks_SERGEANT = []; Ranks_LIEUTENANT = []; Ranks_CAPTAIN = []; Ranks_MAJOR = []; Ranks_COLONEL = []; _playerUID = player getPlayerUID; _found = false; _rank = "PRIVATE"; // default private rank // check to see if our player matches while {!_found} do { if (_playerUID in Ranks_PRIVATE) then { _rank = "PRIVATE"; _found = true; // breaks us out of this while loop }; // ... and so on }; // finally set our players rank! player setRank _rank;
-
Sounds good to me as well. Let me know if you need any help with these classes. I'd like to be a part of this.
-
oh man that is awesome! Glad to see you got it working
-
Just saw this... IDK if this will be helpful for you but it seems to incorporate the distance in the parameters: https://community.bistudio.com/wiki/playSound3D
edit: might not even need a PVEH
-
that... is beyond my expertise. I'm just a simple PVEH coder lol.
You could try the playsound command but... I've never messed with it.
-
just for shits and giggles, can you declare a clientside PVEH just to spit out some text?
// Clientside PVEH (init this in your mission.pbo) if (!isDedicated) then { // expects [] "PVEH_PlaySoundClient" addPublicVariableEventHandler { systemChat "We got our PVEH"; }; };
try placing that in your init.sqf along with your server side one. maybe declare this one first.
-
yeah that is your clientid. If you're running infistar, I believe the file is called admin_start.sqf
-
hmm... try adding _clientID to the private array at the top of the expression. It could be a locality issue? aside from that I'm not really sure.
I'll have to play with the code tonight and see if I can't generate you a sample pbo to play with
-
btw if you are using the stock a3 antihacks, put a file named "skaro.sqf" in your arma3 directory (next to arma3.exe) and you can put code inside of it you want to test out. This only works for clientside code so IDK how helpful it will be for your serverside stuff but you can at least call serverside PVEH's with it.
I think the default keybind is f12 or f11
-
idk you could try setting PVEH_PlaySoundClient = 0;
maybe its angry about an empty array?
-
see if you can throw that in there as that might be causing the error. I looked at the code and nothing pops out at me as a syntax error...
-
can you try throwing in this between the _clientID = and PVEH at line 15/16:
diag_log format["_clientID: %1", _clientID];
this should make it spit out the clientID of whatever the object is... I have a suspicion that it is picking up AI and trying to pass them the PVAR, will probably need to tweak that part a bit.
-
can you pate your TEST/init.sqf ?
-
hahaha this is awesome!
definitely need a more rpg feel to some of the servers.
-
Hey, I saw your tutorial on publiceventhandlers, but I just can't seem to get it well enough to apply it to my script.I want players to hear my player groan when he is playing possum. (from superfunmenu)As it is now, only client can hear the groan.I was hoping you could help me with the basics of getting other players to hear/see my desired sounds/animations.Thanks man.
What you want to do is define a PVEH on the clientside (most likely in the init.sqf or a sqf file called from that) that when fired off, plays a sound.
How you fire it off is the tricky part as you probably only want people in say a 30m radius to hear the sound. You could be lazy and just fire it off to everyone.
So here's how I would do it quick and dirty. Note, there is probably a better way to do this but its monday...
So, you need 2 pveh.
1. Serverside PVEH to get our call from our player and send to other players
2. Clientside PVEH to actually play the sound.
// Serverside PVEH (Init this in your server.pbo) if (isDedicated) then { // expects [Player,Radius] "PVEH_PlaySoundServer" addPublicVariableEventHandler { private["_packet", "_sender", "_radius", "_playersToSend"]; _packet = _this select 1; _sender = _packet select 0; _radius = _packet select 1; _playersToSend = nearestObjects [_sender, ["CAmanBase"], _radius]; { _clientID = owner _x; PVEH_PlaySoundClient = []; _clientID publicVaribleClient "PVEH_PlaySoundClient"; } forEach _playersToSend; }; }; // Clientside PVEH (init this in your mission.pbo) if (!isDedicated) then { // expects [] "PVEH_PlaySoundClient" addPublicVariableEventHandler { // play sound code goes here }; };
Now please note, that the "get players within raidus" code is probably inefficient and probably buggy. I'm sure there is a better way to do this and you are free to look into that part, I just wrote this up real quick to give you an idea on how you can do this.
edit: sorry I forgot, somewhere in your code to kick this off, you will need to call a publicVariableServer command to kick it off like this:
PVEH_PlaySoundServer = [player,30]; // [player,radius] publicVariableServer "PVEH_PlaySoundServer";
-
just for clarification, when you start bec, shouldn't it do something other than close? this is what I'm seeing:
Restricted Building Areas
in Scripting
Posted
yep, merged it over and am good to go!
Thanks again, guys!