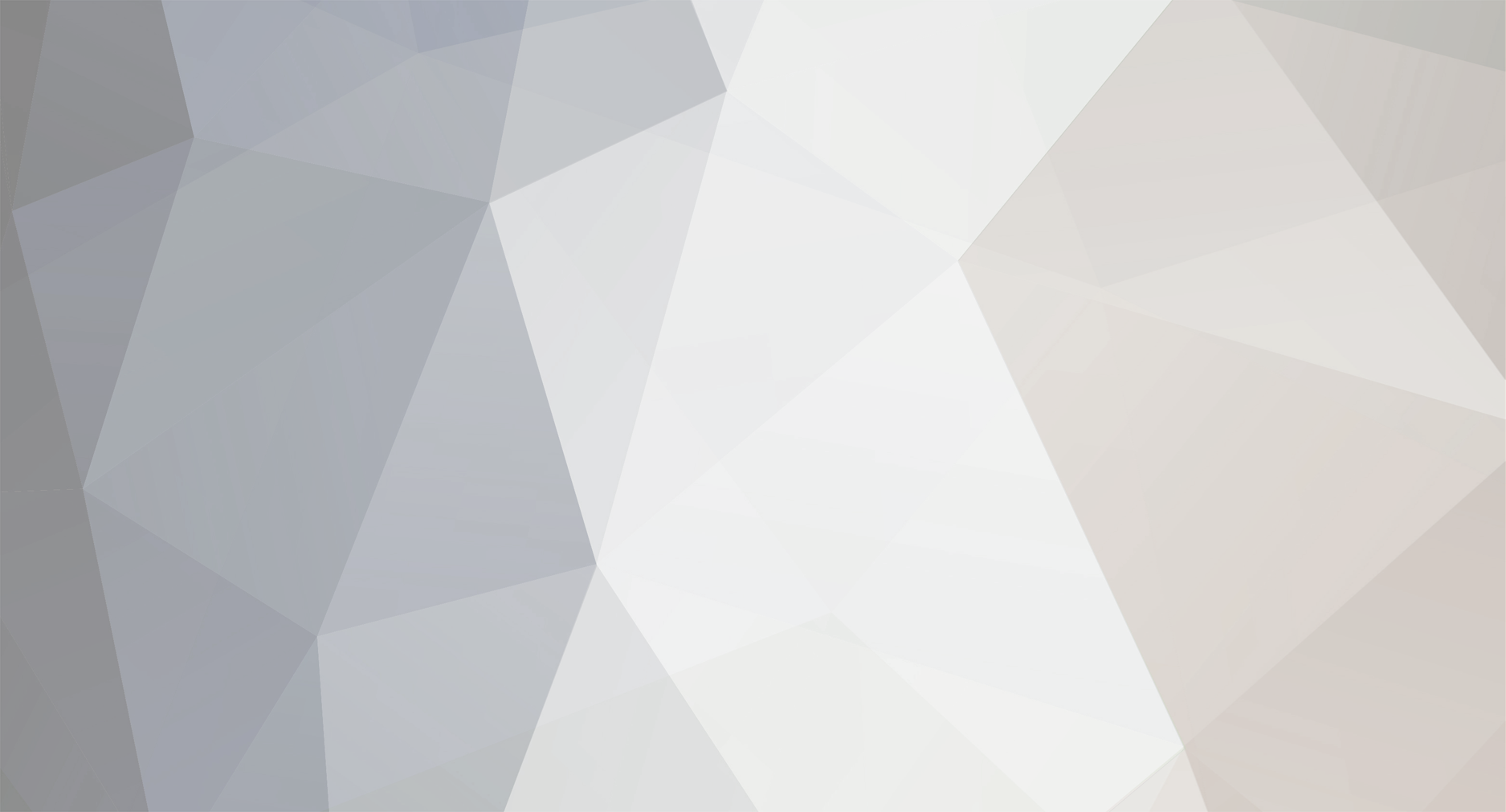
bucki
-
Posts
5 -
Joined
-
Last visited
Content Type
Profiles
Forums
Articles
Posts posted by bucki
-
-
thanks for this great work, it´s really good work.
runs up to one thing for me.
although ai wear grenate launcher on his back, but they don t use them.
i tested with different cars and helicopter and have no solution, where is my mistake.
bucki
-
Hello,
i have a Problem,
. using following Mission Systems
WAI, Blackeagle, A3a1
Epoch 0.3.0.2 Altis
After a Player revive otherone, ai´s can t make damage on Player. Player vs Player can kill.
and sapper can kill too.
anyone have an issue for this problem?
Thanks for Help bucki
-
thanx hardened,
for you help.
-
hello,
i ve build in 3d Editor rooms with doors.
the Player should upgrate the doors ingame with a combination lock.
Player get the message that they cant. I think the issue is, that the doors are not in database and build as Mission file?
i think i can t fix the Problem or have anyone an solution?
thanx for you help
blckeagls' AI Mission - Version 2.0.2 Release (1/2/2015)
in AI Missions
Posted
I think i found the problem.
you cant put the rockets for granate launcher into a vest, You need backpack for this.
hope i make the changes correct. on my testserver it runs.
and sorry for my bad english.